Android
[Android Studio] 안드로이드 스튜디오 Java Recycler View 만들기
파송송
2022. 8. 18. 21:06
728x90
1. Build.gradle 수정
implementation 'com.android.support:recyclerview-v7:28.0.0'
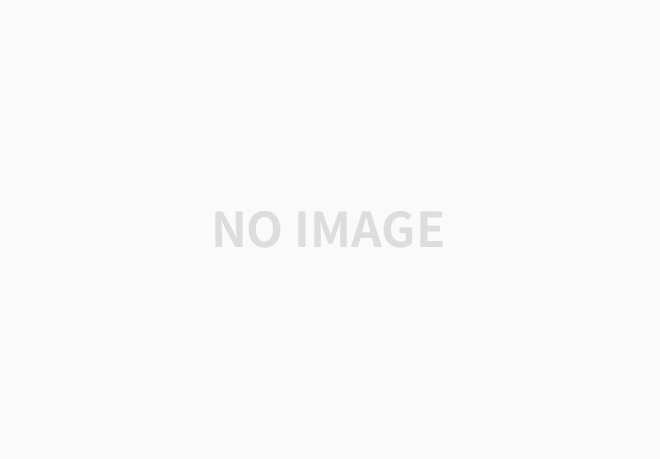
https://pasongsong.tistory.com/123
[Android Studio] Version 28 (intended for Android Pie and below) is the last version of the legacy support library, so we recomm
Refactor > Migrate to AndroidX 체크해서 백업 해주고 Migrate 클릭 자동으로 생성해줌 굿
pasongsong.tistory.com
2. Item layout 구성
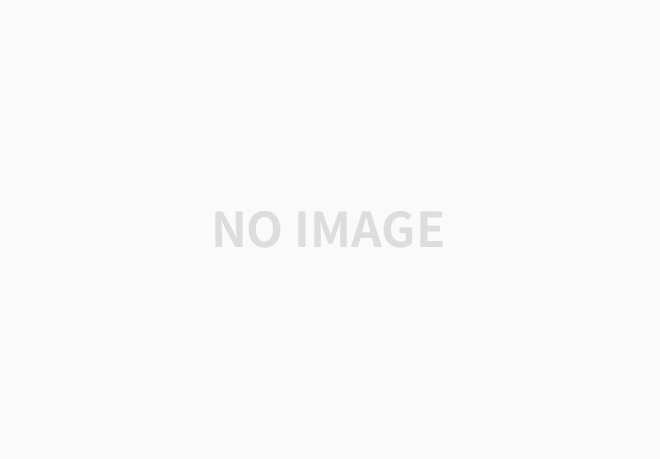
하나 만들어 줍니다.
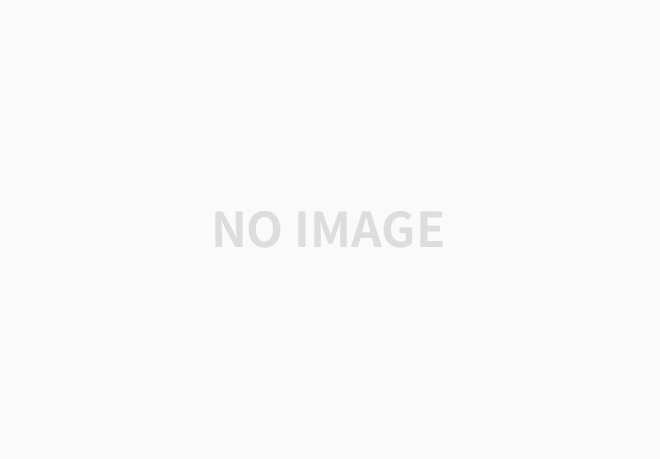
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<ImageView
android:id="@+id/IV_food"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_marginBottom="5dp"
app:layout_constraintBottom_toTopOf="@+id/BTN_insert_food"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.0"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.0"
tools:srcCompat="@tools:sample/avatars" />
<Button
android:id="@+id/BTN_insert_food"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="Button"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
tools:layout_editor_absoluteY="319dp" />
</androidx.constraintlayout.widget.ConstraintLayout>
3. Class 만들기
package com.example.k_food_application;
public class One_food {
int food_resourceId;
public One_food(int food_resourceId){
this.food_resourceId = food_resourceId;
}
public int getFood_resourceId(){
return food_resourceId;
}
public void setFood_resourceId(int food_resourceId){
this.food_resourceId = food_resourceId;
}
}
4. Adapter 구현
https://developer.android.com/guide/topics/ui/layout/recyclerview?hl=ko
RecyclerView로 동적 목록 만들기 | Android 개발자 | Android Developers
RecyclerView로 동적 목록 만들기 Android Jetpack의 구성요소 RecyclerView를 사용하면 대량의 데이터 세트를 효율적으로 표시할 수 있습니다. 개발자가 데이터를 제공하고 각 항목의 모양을 정의하면 R
developer.android.com
package com.example.k_food_application;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ImageView;
import androidx.annotation.NonNull;
import androidx.recyclerview.widget.RecyclerView;
import java.util.ArrayList;
public class DayMealAdapter extends RecyclerView.Adapter<DayMealAdapter.ViewHolder> {
private ArrayList<One_food> food_list;
/**
* Provide a reference to the type of views that you are using
* (custom ViewHolder).
*/
@NonNull
@Override
public DayMealAdapter.ViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext()).inflate(R.layout.one_food, parent, false);
return new ViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull DayMealAdapter.ViewHolder holder, int position) {
holder.onBind(food_list.get(position));
}
public void setFoodList(ArrayList<One_food> list){
this.food_list = list;
notifyDataSetChanged();
}
@Override
public int getItemCount() {
return food_list.size();
}
class ViewHolder extends RecyclerView.ViewHolder {
ImageView food_img;
public ViewHolder(@NonNull View itemView) {
super(itemView);
food_img = (ImageView) itemView.findViewById(R.id.IV_food);
}
void onBind(One_food item){
food_img.setImageResource(item.getFood_resourceId());
}
}
}
5. Main 수정
//recycler View 연결
RecyclerView one_food = (RecyclerView) findViewById(R.id.Recyc_food);
DayMealAdapter one_food_Adapter = new DayMealAdapter();
one_food.setAdapter(one_food_Adapter);
one_food.setLayoutManager(new LinearLayoutManager(this));
ArrayList<One_food> meal_items = new ArrayList<>();
meal_items.add(new One_food(R.drawable.ic_camera));
one_food_Adapter.setFoodList(meal_items);
728x90